Browser-based automation with Step and Selenium
This article defines three Keywords which will be used in browser-based automation scenarios, using Step and Selenium, as general drivers.
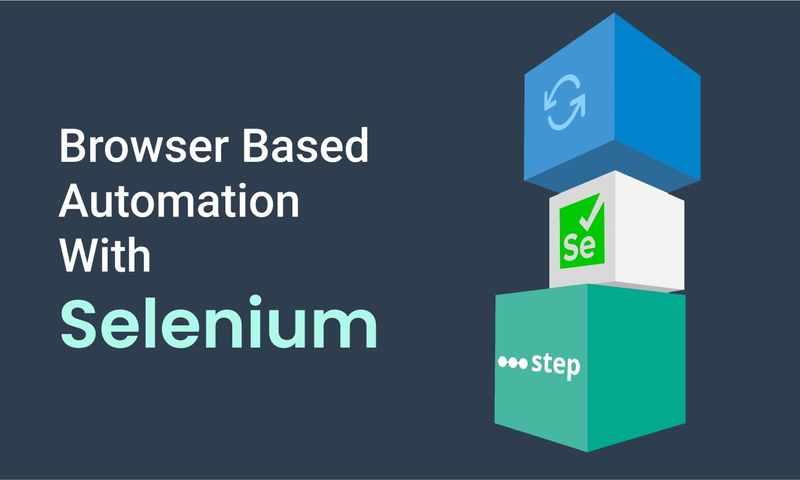
The Selenium framework provides the ability to automate browser actions (browsing, clicking, filling out forms, etc.) and is commonly used for automation testing. You can find more information on the official Selenium website. Examples of Selenium Keywords are available on GitHub.
Preface
In this Selenium tutorial, we will define 3 Keywords:
- OpenChrome, which will be used to create the driver object and the driver wrapper, and pass the driver wrapper to the step’s session
- NavigateTo, which will be used to navigate to an url passed via the input
- CloseChrome, which will be used to explicitly close the driver
The following information provides context for and supplements this tutorial. Please review this information and follow all configurations before beginning step one of the tutorial.
Maven Settings
Using Selenium as a library
Selenium requires several dependencies, as can be seen in the following “pom.xml” file:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>my.project.example</groupId>
<artifactId>selenium-dependencies</artifactId>
<packaging>jar</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<selenium.version>3.14.0
</properties>
<dependencies>
<dependency>
<groupId>ch.exense.step</groupId>
<artifactId>step-api-keyword</artifactId>
<version>${step-api.version}</version>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-api</artifactId>
<version>${selenium.version}</version>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>${selenium.version}</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.1</version>
<executions>
<execution>
<phase>package
<goals>
<goal>shade</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
Webdriver Management
When it comes to WebDriver management, rather than imposing a particular lifecycle management approach onto the developer, instead we let them decide how and when they want to store the driver.
We strongly recommend the following best practices to keep WebDriver management smooth and simple:
- For beginners who are using Selenium or step on their first attempt, we recommend packing the whole scenario, including driver creation and destruction, in a single keyword, and then experimenting with the API until they’ve built a basic test plan that does what they expect
- For more advanced users or load testers who need to have more control over driver creation and destruction and be independent of existing workflows, we encourage you to:
- Create explicit Keywords for the creation and destruction of the driver and implement the rest of the workflow’s logic in separate keywords
- Work in “stateful” mode by introducing a Session object in your test plan, which will guarantee at runtime that the context of each thread/virtual user is maintained and isolated from the others
- This will allow you to add a for loop object and have your users iterate in a certain scenario without destroying the driver (and if you wish, without logging out)
- Attach the driver object to step’s session object, which is managed internally by step and will allow for the “business keywords” to use a properly initialized driver (see example below)
- Wrap the driver in a class which implements Closable to help step clean up in error scenarios (see below as well)
- Lastly, in order to achieve maximum Keyword granularity (and reduce maintenance efforts), especially in environments involving many partially overlapping workflows, we recommend separating each logical step of the workflow in distinct Keywords and passing the driver between keywords
Driver Wrapper Class
Let us take a look at our DriverWrapper class in order to understand how the process gets setup:
public class DriverWrapper implements Closeable {
// Our driver object
final WebDriver driver;
// Constructor assigning the given driver object to the local one
public DriverWrapper(WebDriver driver) {
this.driver = driver;
}
// Implementing the Closeable interface requires to override the close() method, this is where we destroy the driver object
@Override
public void close() throws IOException { driver.quit();
}
}
Tutorial
- Set the Type to Java and add the generated jar file as a library:
- Navigate to the url passed in Input, and check if one of the h1 tags contains the text “Discover”. Here is the code for this:
@Keyword(name="Selenium_Example")
public void Selenium_Example() {
// In this example we will use Chrome, so we explicitly set the location of its web driver
System.setProperty("webdriver.chrome.driver", "C:\\Tools\\chromedriver.exe");
// Create a new driver object in order to execute actions
ChromeDriver chrome = new ChromeDriver();
// Get the url value from the input
String homeUrl = input.getString("url");
// Navigate to the url
chrome.navigate().to(homeUrl);
// Look for an h1 tag containing the text "Discover"
chrome.findElement(By.xpath("//h1[contains(text(),'Discover')]"));
}
- Run this code:
public class SeleniumExample extends AbstractKeyword {
// Private method in order to get the WebDriver from the DriverWrapper object
final WebDriver getDriver() {
return session.get(DriverWrapper.class).driver;
}
@Keyword(name="OpenChrome")
public void OpenChrome() throws Exception {
// Set explicitly web driver location
System.setProperty("webdriver.chrome.driver", "C:\\Tools\\chromedriver.exe");
// Create the driver object
final WebDriver driver = new ChromeDriver();
// Tell the driver to timeout after 10 seconds
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Put the DriverWrapper object into step's session
session.put(new DriverWrapper(driver));
}
@Keyword(name="NavigateTo")
public void NavigateTo() throws Exception {
// Check if the Input contains the url
if(input.containsKey("url")) {
// Get the driver from step's session
final WebDriver driver = getDriver();
// Navigate to the provided url
driver.navigate().to(input.get("url").toString());
} else {
output.setError("Input parameter 'url' not defined");
}
}
@Keyword(name="CloseChrome")
public void CloseChrome() throws Exception { // Get the driver from step's session
final WebDriver driver = getDriver();
// Close explicitly the driver
driver.quit();
}
}
- Now deploy and create our Keywords:
- OpenChrome, which will be used to create the driver object and the driver wrapper, and pass the driver wrapper to the step’s session
- NavigateTo, which will be used to navigate to an url passed via the input
- CloseChrome, which will be used to explicitly close the driver
- Create a test plan using our Keywords:
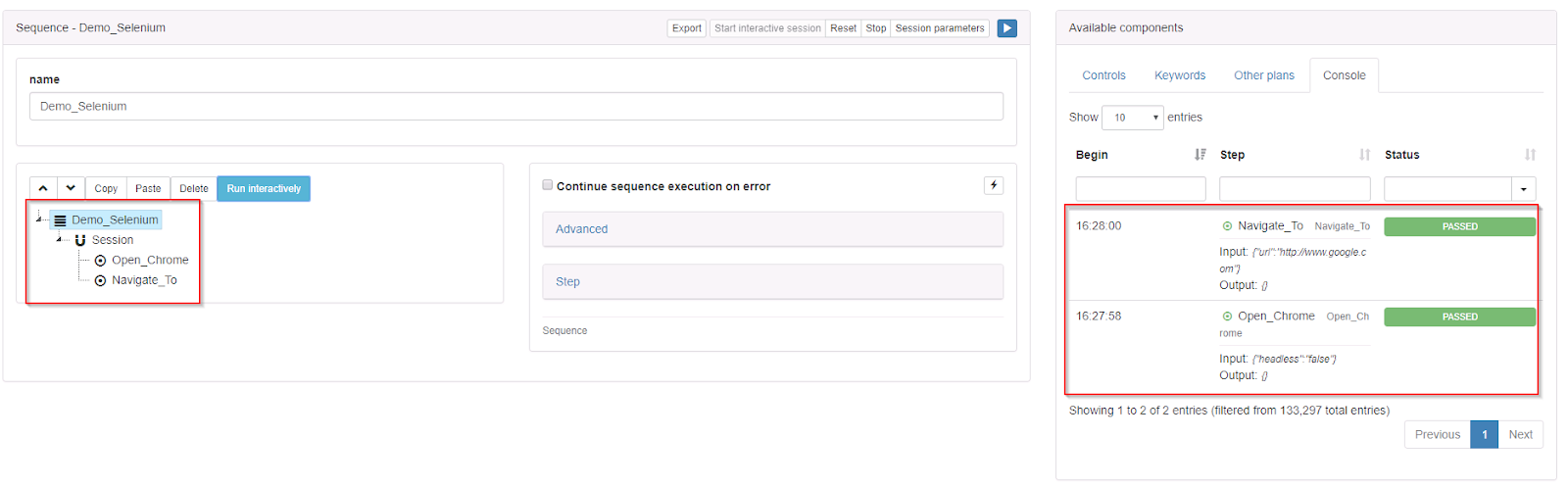
Summary: This article defines three Keywords (OpenChrome, NavigateTo, and CloseChrome) which will be used in browser-based automation scenarios, using Step and Selenium, as general drivers and navigators.
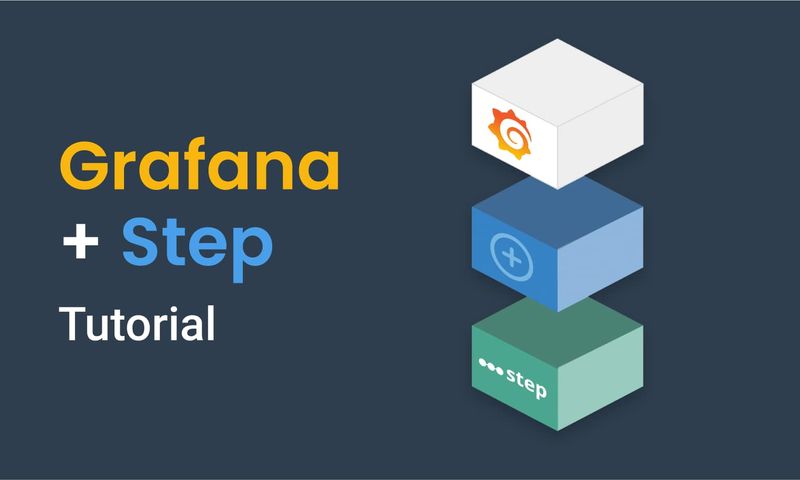
This article demonstrates how to connect Grafana to data generated by Step.
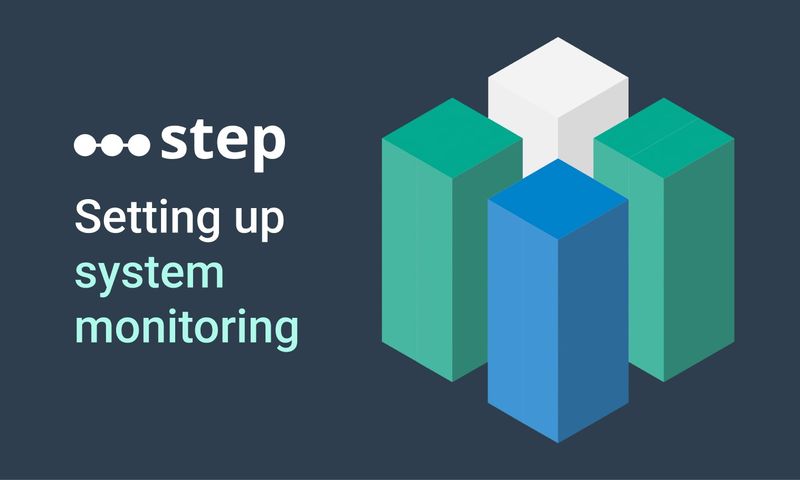
This article demonstrates how to set up distributed system monitoring using Keyword executions, and analyze the results as measurements.
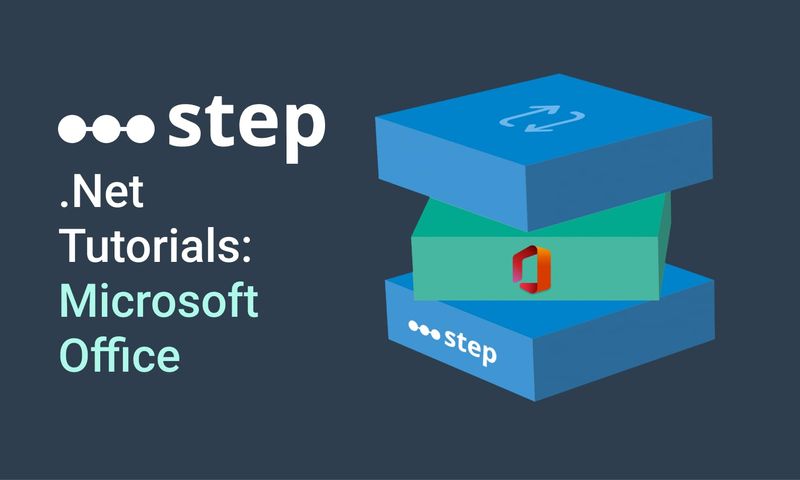
This tutorial demonstrates how to automate interaction with Microsoft Office applications using the Office Interop Assembly.
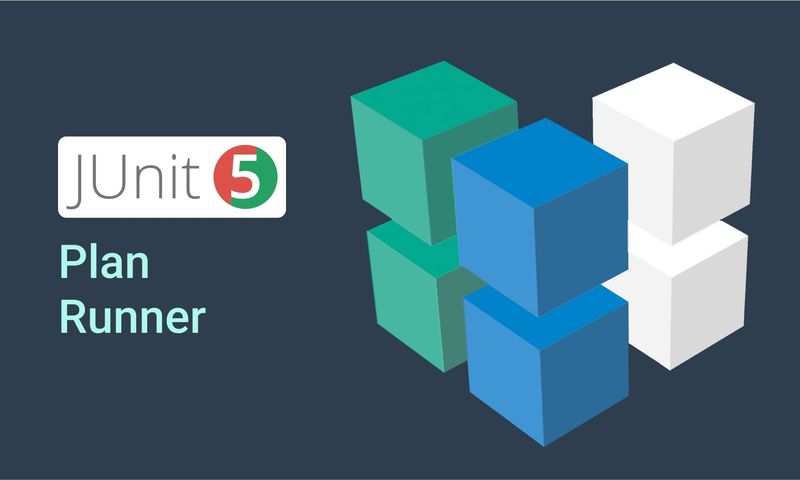
This article provides documentation for how to integrate JUnit tests into Step.
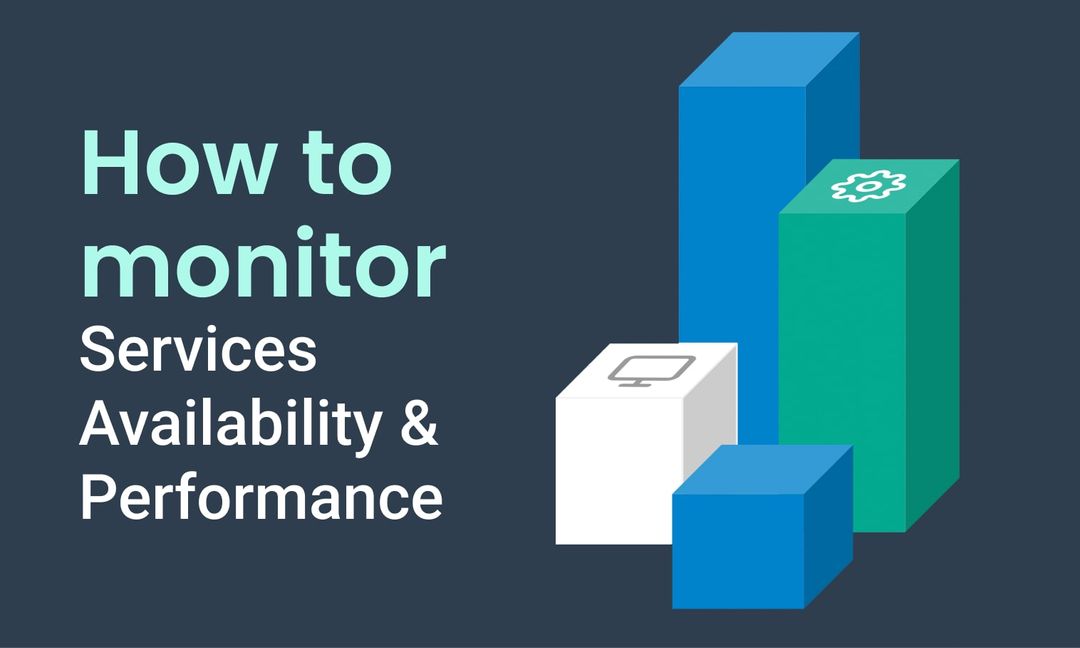
This tutorial demonstrates how Step can be used to monitor services, availability and performance metrics.
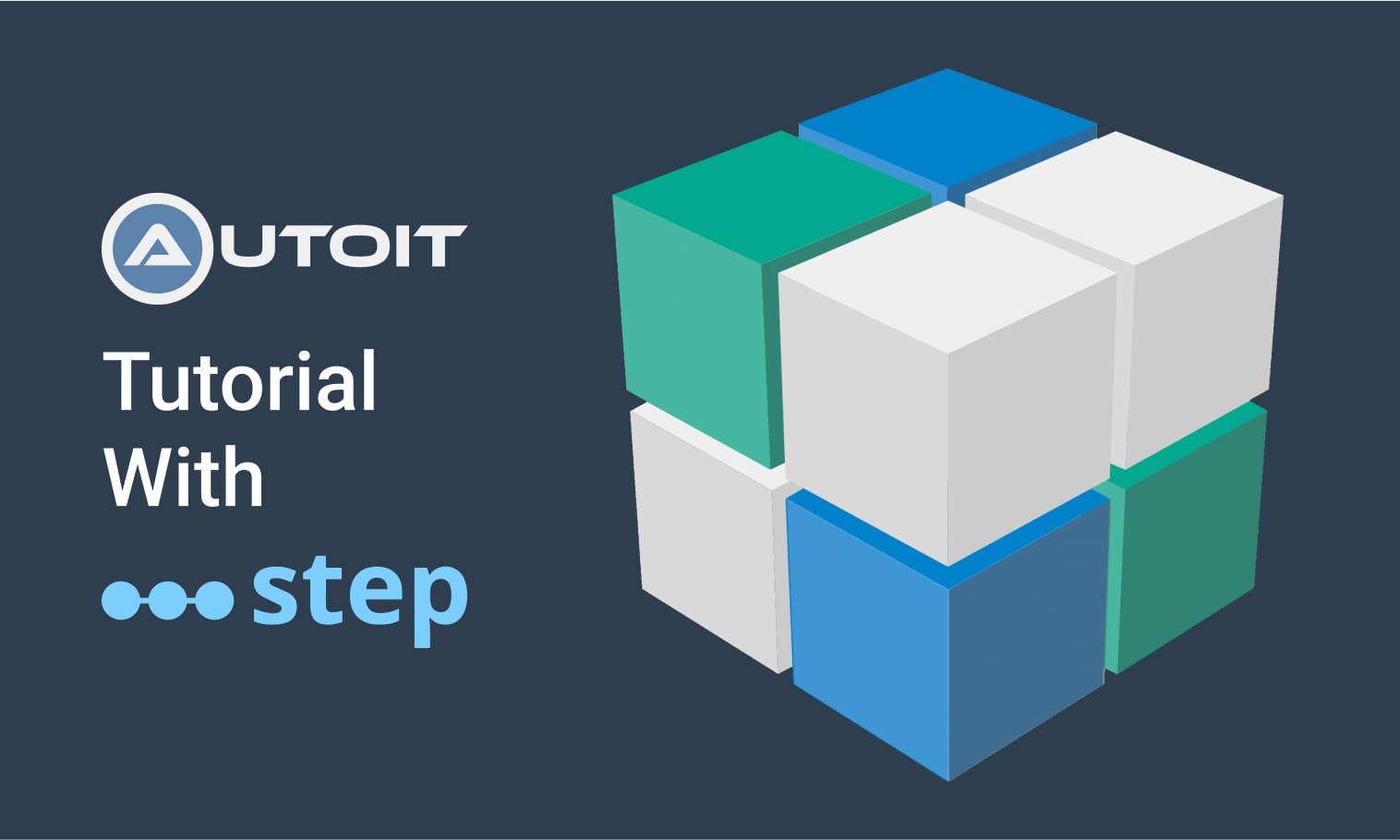
This tutorial demonstrates how to utilize the AutoIt C# binding to automate interactions with Windows applications.
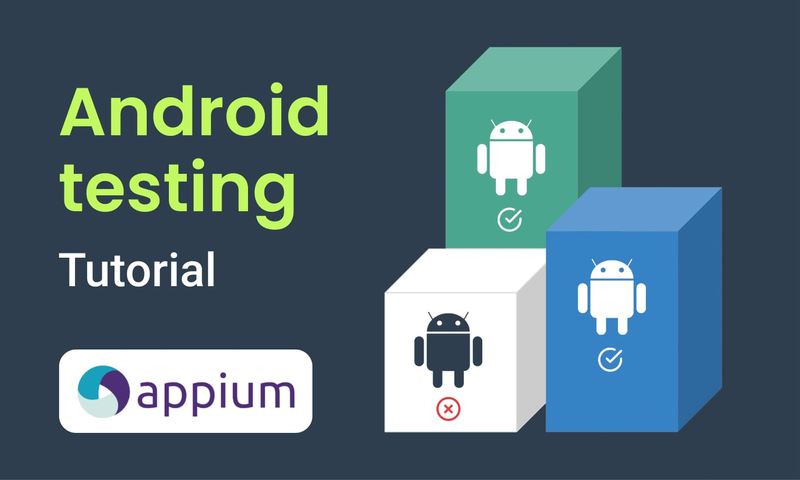
This article demonstrates the automation of mobile applications on Android using the Appium framework.
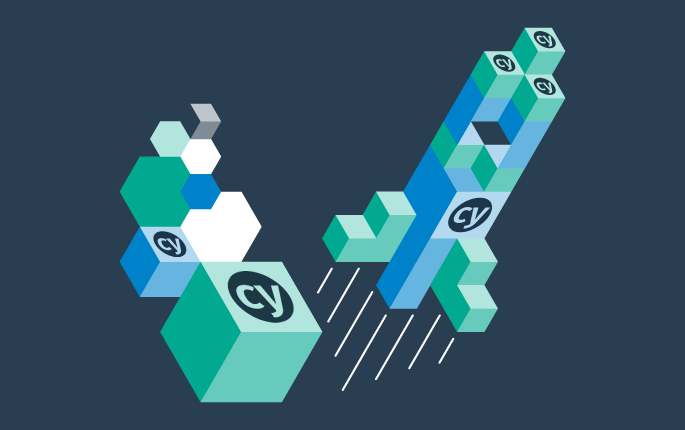
This tutorial shows you how to efficiently set up a browser-based load test using existing Cypress tests in the Step automation platform.
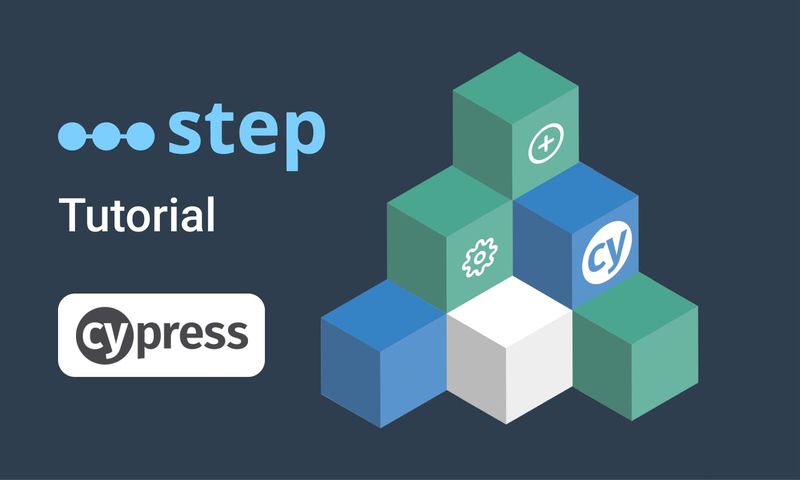
In this short tutorial, we show how to quickly implement a simple browser-based load test based on Cypress scripts in Step.
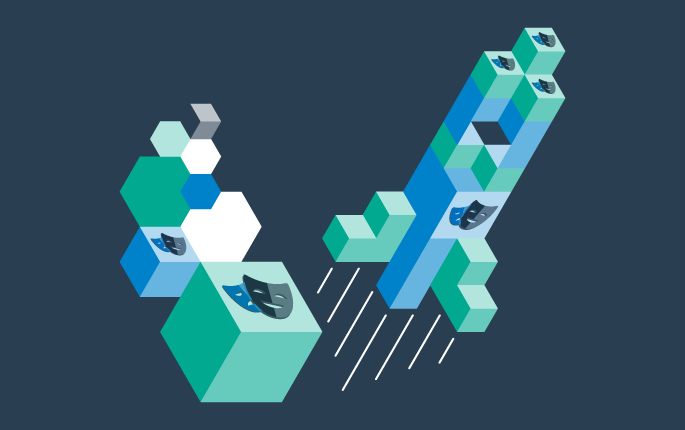
This tutorial shows you how to efficiently set up a browser-based load test using existing Playwright tests in the Step automation platform.
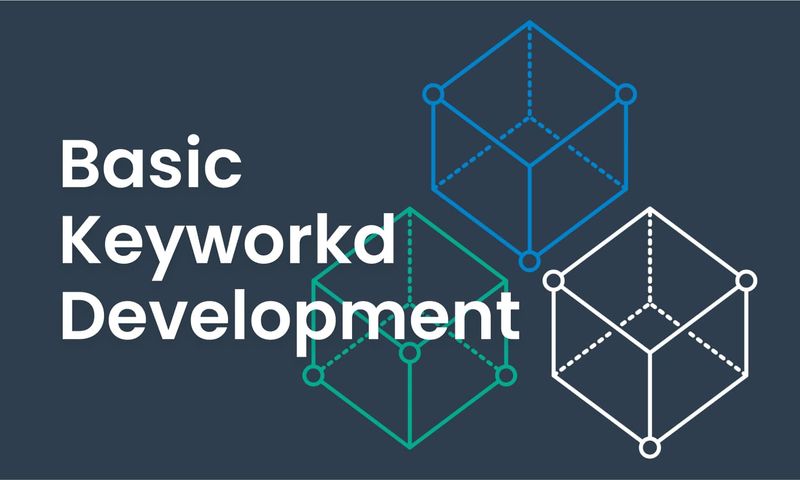
This article explains Keywords in Step and demonstrates how to create simple ones.
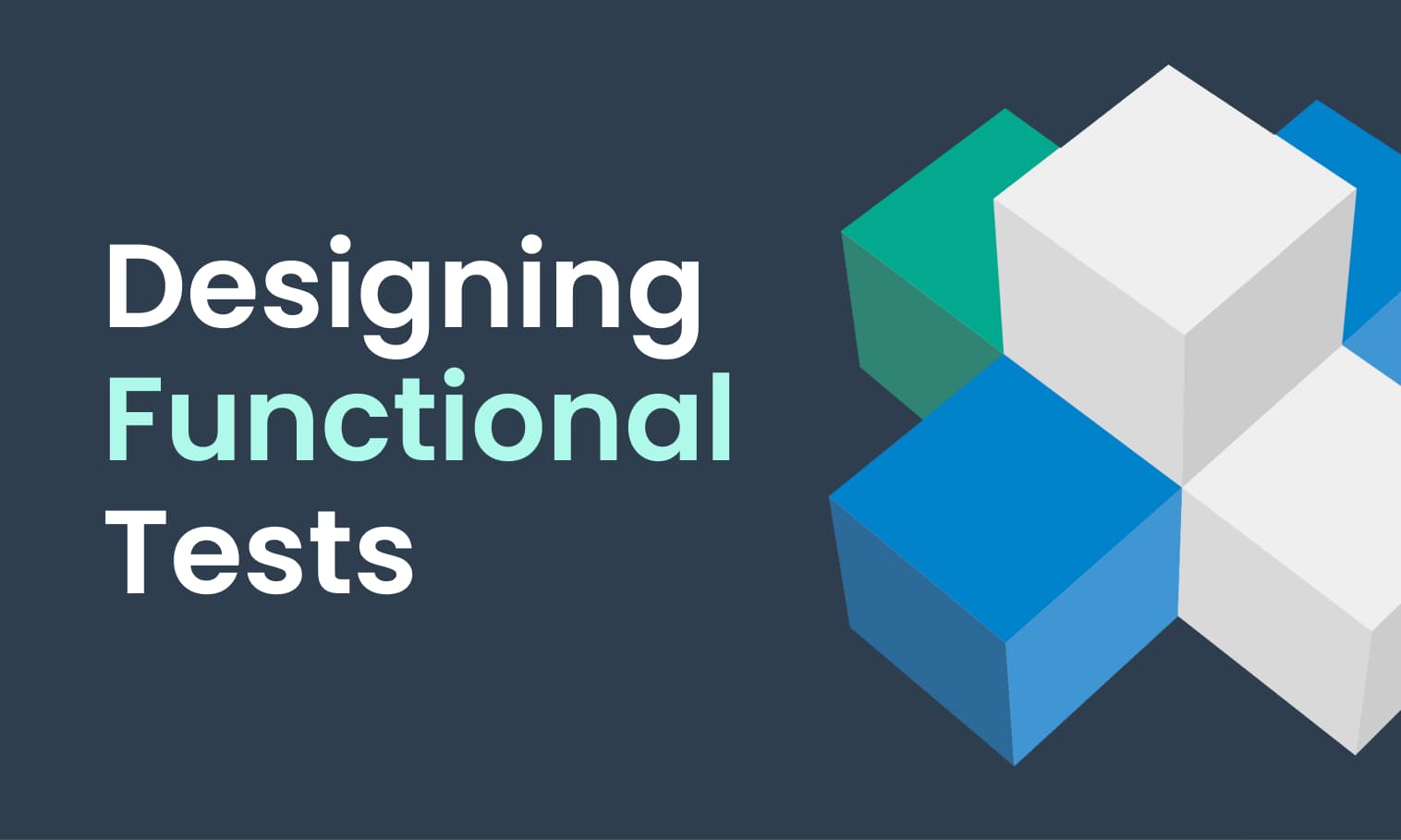
This tutorial demonstrates the design, execution, and analysis of functional tests using the web interface of Step.
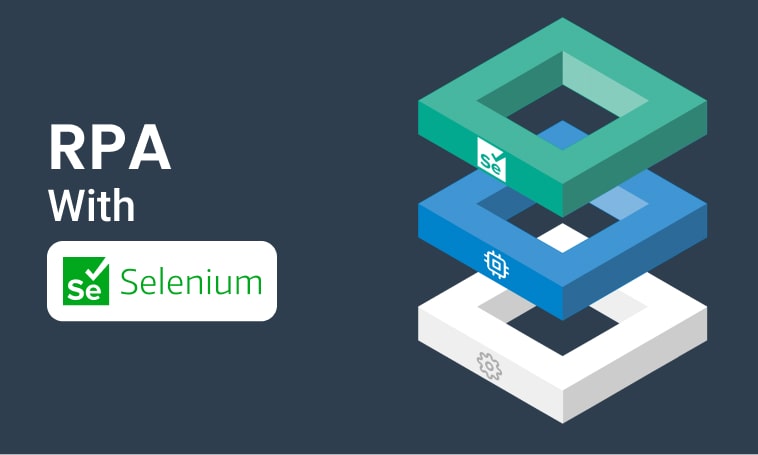
This tutorial will demonstrate how to use Step and Selenium to automate various browser tasks.
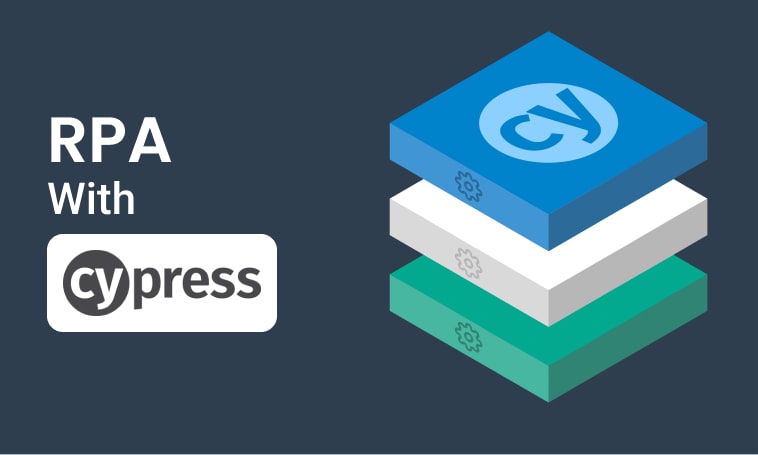
This tutorial demonstrates how to use Step and Cypress to automate various browser tasks.
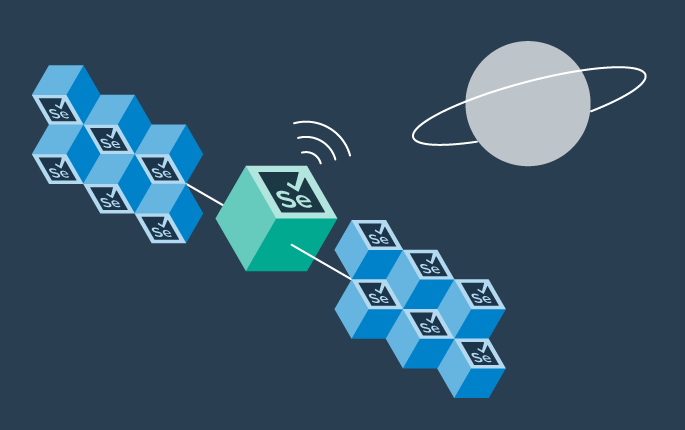
This tutorial demonstrates how Selenium automation tests can be turned into full synthetic monitoring using Step.
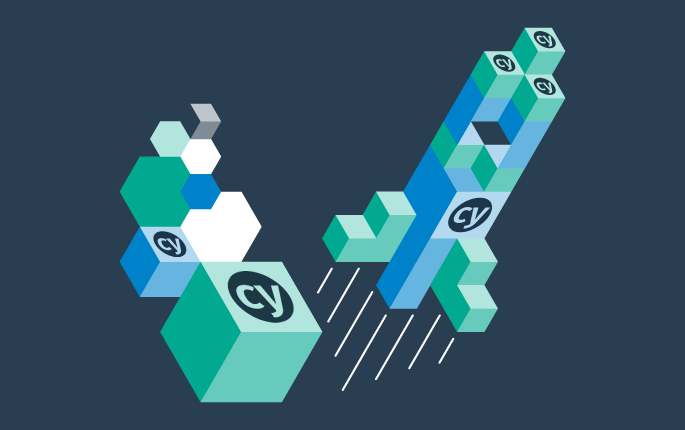
In this tutorial, we'll show you how to easily set up and run a browser-based load test with Step's wizard, using your existing Cypress tests, on the Step automation platform.
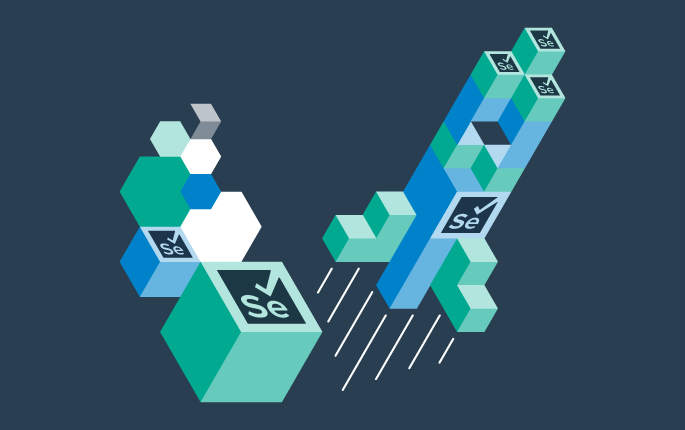
This tutorial shows you how to efficiently set up a browser-based load test using existing Selenium tests in the Step automation platform.
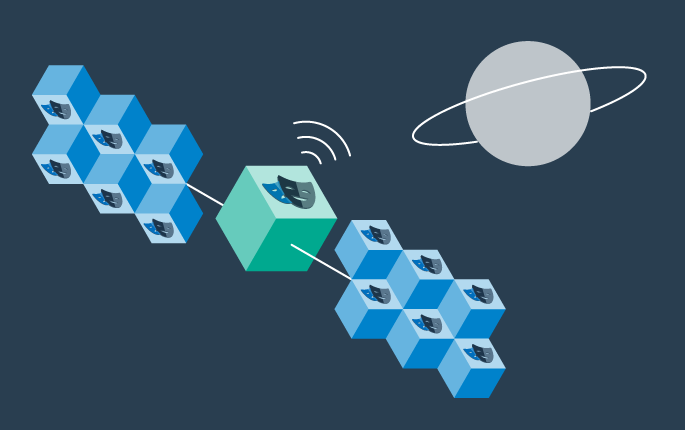
This tutorial demonstrates how Playwright automation tests can be turned into full synthetic monitoring using Step.

This tutorial demonstrates how Cypress automation tests can be turned into full synthetic monitoring using Step.
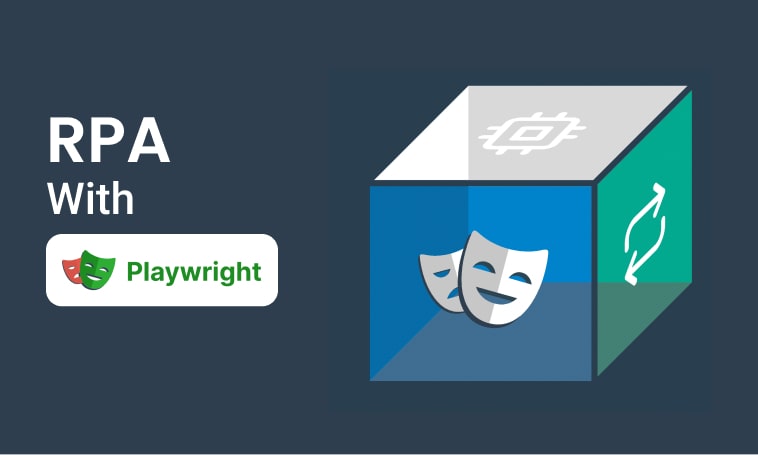
This tutorial will demonstrate how to use Step and Playwright to automate various browser tasks.
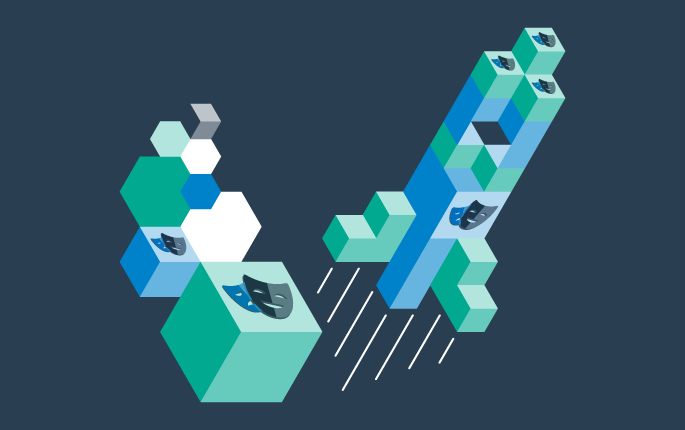
In this tutorial, we'll show you how to easily set up and run a browser-based load test with Step's wizard, using your existing Playwright tests, on the Step automation platform.
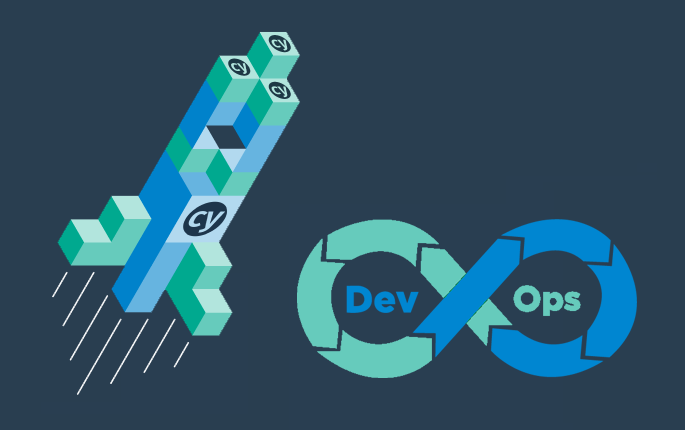
Learn how to quickly set up continuous browser-based load testing using Cypress tests in your DevOps pipeline
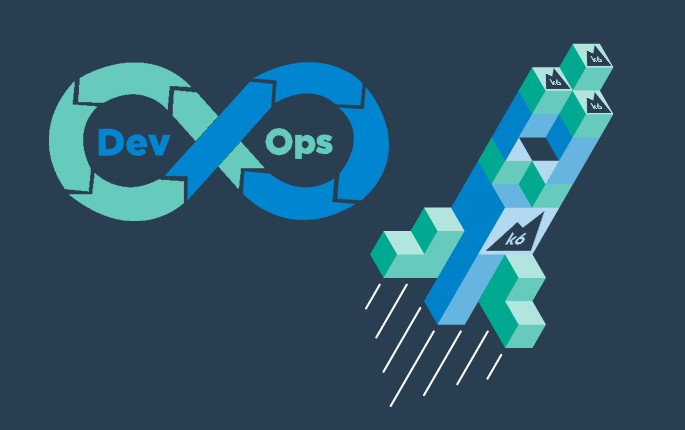
Quickly integrate K6 based load-tests in your DevOps workflow
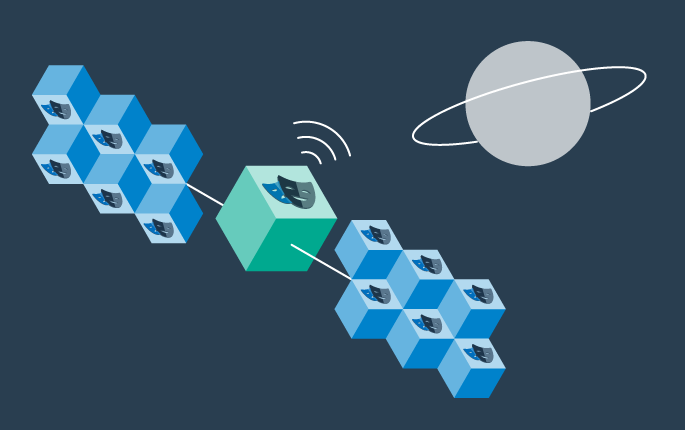
This tutorial demonstrates how Playwright tests can be reused for synthetic monitoring of a productive environment in a DevOps workflow
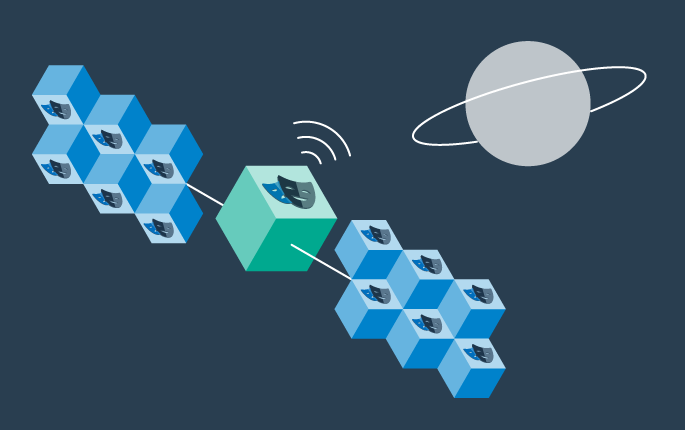
This tutorial demonstrates how Playwright tests can be reused for synthetic monitoring of a productive environment in a DevOps workflow
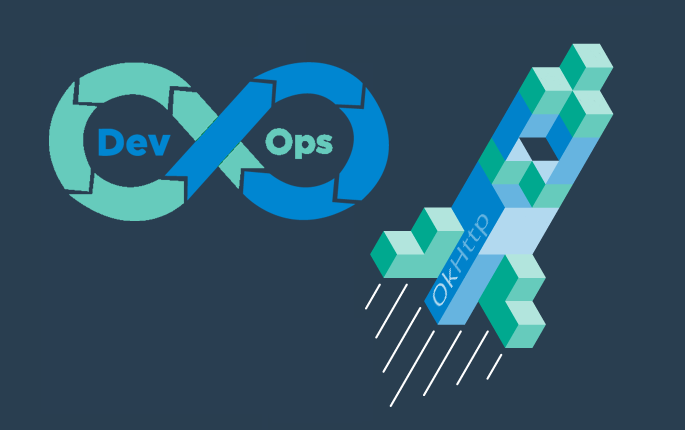
In this tutorial you'll learn how to quickly set up a protocol-based load test with okhttp

Learn how to set up continuous end-to-end testing across several applications based on Playwright tests in your DevOps pipeline using Step

Learn how to quickly set up continuous browser-based load testing using Playwright tests in your DevOps pipeline
Want to hear our latest updates about automation?
Don't miss out on our regular blog posts - Subscribe now!